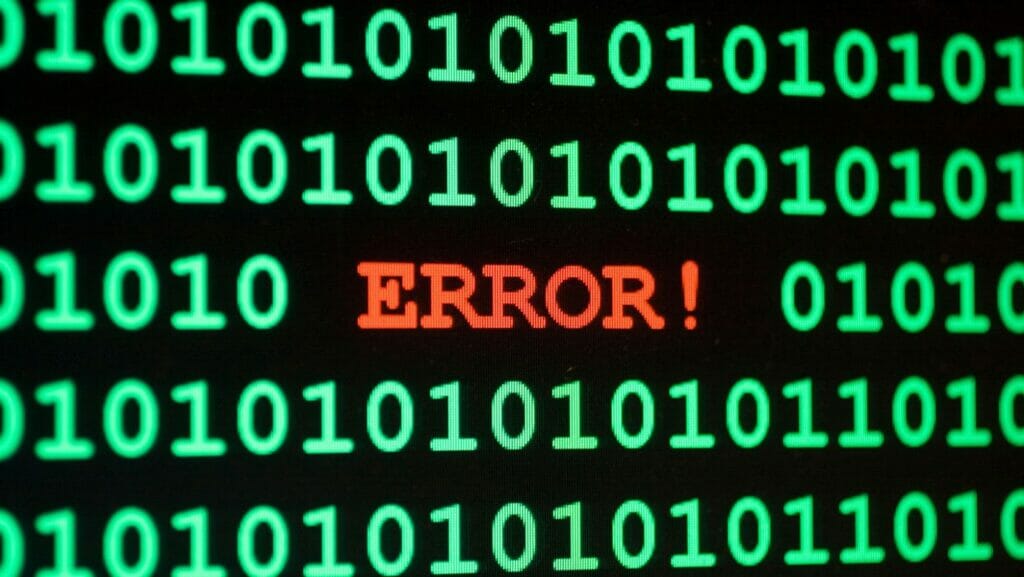
Error coding is a method of detecting and correcting errors in data transmission, storage, and retrieval. There are several examples of error coding techniques that are commonly used in modern computing and telecommunications systems.
One example of error coding is the cyclic redundancy check (CRC), which works by adding an extra set of bits to a data packet that allows the receiver to check for errors caused by noise or other transmission problems.
Another example is forward error correction (FEC), which involves adding redundant information to a data stream that allows errors to be corrected without the need for retransmission. Parity checking, Hamming codes, and Reed-Solomon codes are other examples of error coding techniques used in various applications.
By using error coding techniques, data can be transmitted and stored more reliably, with a lower risk of corruption, loss, or data breaches.
Pro tip: Error correction techniques are an essential part of modern data communication and storage systems. By understanding how error coding works, you can design more robust systems and troubleshoot problems more effectively.
Introduction to Error Coding
Error coding is a rigorous form of programming that helps developers identify and fix bugs in code. A common type of error coding is static analysis, which is a type of analysis that looks at the code being written before it is compiled and run. This can help developers spot issues in the code and make corrections before the code goes into production.
In this article, we will discuss some of the common examples of error coding.
Sorry, something unexpected happened. try again. (unknown error (empty response) (#1.1357045))
Error coding is a method used to detect and correct errors that may occur during data transmission or data storage. It involves adding extra bits of information to the data that enable the receiver to identify and correct any errors that occur.
One example of error coding is the parity check, which involves adding a single parity bit to a group of bits to ensure that the number of bits with a value of one in the group is always odd or even. If a bit is lost or changed during transmission, the receiver can compare the parity bit to the remaining bits and identify the error. There are many types of error codes, each with its own strengths and weaknesses. Choosing the right error code for a particular use case requires an understanding of the data being transmitted or stored and the potential sources of errors.
Pro tip:Â Error coding is an essential technique used in many modern communication systems and storage devices to ensure data integrity.
Ocurrió algo inesperado. vuelve a intentarlo. (unknown error (empty response) (#1.1357045))
Error coding is an essential aspect of modern communication and computing systems. It involves the use of mathematical algorithms to detect and correct errors that may occur during data transmission or storage.
One popular example of error coding is the checksum method, where the sender adds up the numerical values of the data being transmitted and sends the result along with the data. The receiver performs the same calculation and compares the results. If there is a mismatch, an error has occurred, and the data can be re-transmitted or corrected.
Other examples of error coding techniques include Hamming codes, Reed-Solomon codes, and convolutional codes.
Without error coding, the data transmitted over a channel could be corrupt, leading to a range of problems, from minor glitches to security breaches or catastrophic system failures. By employing error coding, we ensure that data is transmitted efficiently and accurately, making it an integral part of modern communication and computing systems.
Error During Xslt Transformation an Unknown Error Has Occurred Drac
Errors are a common occurrence in coding and can lead to issues such as bugs, crashes, malfunctions, and compromised security. Here are the main types of errors in coding:
Syntax errors: These occur when you violate the syntax rules of a programming language by making mistakes in your code’s structure and keywords. For instance, using a semicolon instead of a colon in a python loop.
Runtime errors: These happen when your code runs, and it faces some challenges that prevent it from executing correctly.
Logical errors: These errors are hard to detect and occur when your code produces unexpected results based on the input data provided. The issue is not that the code results don’t comply with the syntax, but the code might be executing the wrong branch based on the input parameters.
By understanding the types of errors in coding, programmers can accurately diagnose and fix them while improving their skills.
Dashlane Unknown Error 2065
Error coding is a necessary part of software development, as it helps developers identify and fix errors in their code. As such, it is important to understand what exactly it is and what examples of it exist.
In this article, we will discuss the definition of error coding and provide some examples of it.
Syntax Errors
Syntax errors are common in programming and occur when the code violates the rules of the programming language. It is essential to identify and resolve syntax errors before running the program. Here are a few examples of error coding:
Misspelled or misplaced keywords: Spelling mistakes, missing or misplaced punctuations, and keywords such as “if” or “else” used incorrectly can cause syntax errors.
Incorrect indentation: Many programming languages require indentation to indicate code blocks. Incorrect indentation can result in syntax errors.
Missing or incorrect brackets: Many programming languages use brackets to enclose code blocks. Missing or incorrect brackets can cause syntax errors and prevent the program from running.
Typos in variable or function names: Typos in variable or function names can lead to syntax errors and can be challenging to debug.
Unused or undefined variables: Using a variable that is either undefined or not used can cause syntax errors.
Pro Tip:Â Use a code editor with auto-complete features and syntax highlighting to avoid syntax errors.
Could Not Retrieve Targeting Information: Unknown Error (Empty Response) (#1.1357045)
Syntax errors are common mistakes in programming that occur when the code contains incorrect syntax or misspelt keywords. These errors prevent the program from running and can be frustrating for beginner programmers. Examples of syntax errors include unclosed quotes, misplaced symbols, misspelt variable names, or incorrect indentation.
For instance, an example of error coding can be:
if x = 5: # should be if x == 5:
print(‘x is equal to 5.’)
TypeError: ‘=’ is an assignment operator, ‘==’ should be used for comparison
Another example could be:
print(Hello, World!) # should be print(‘Hello, World!’)
SyntaxError: invalid syntax
To avoid syntax errors, it’s essential to proofread your code, use professional tools or IDE that highlight issues and follow the syntax rules of the programming language you’re working with.
Unknown Error 0xe80004
Syntax errors are common mistakes made by programmers when writing code. Here are a few ways to identify syntax errors:
Check for error messages: Most compiler or interpreter programs will display error messages that specify the type and location of the syntax error.
Look for typos: Syntax errors can occur due to simple typos, such as mistyping keywords or not closing parentheses or quotes.
Check for incorrect indentation: In programming languages that rely on indentation as a way to organise blocks of code, incorrect indentation can lead to syntax errors.
Pro Tip:Â To avoid syntax errors, it’s important to write clean and organised code, use a reliable text editor or IDE, and test your code regularly to catch errors early on.
Runtime Errors
At times, software programs encounter errors that cause them to malfunction or even crash. Runtime errors are one of the most common types of error coding. Some examples of runtime errors include unexpected user input, missing files or libraries, and invalid memory addresses.
A common example of a runtime error is the “divide by zero” error, which occurs when a program attempts to divide a number by zero, resulting in an undefined value. Another example is “stack overflow” error, which happens when a program runs out of memory allocated to it, causing the program to crash.
Proper testing and debugging techniques can help identify and fix these errors in software programs.
Definition and Examples of Runtime Errors
A runtime error is an error that occurs during the execution of a program, causing it to prematurely terminate or produce unexpected results. Here are some examples of runtime errors:
Divide by zero: This happens when a program tries to divide a number by zero, which is undefined and results in an error.
Null pointer dereference: This occurs when a program tries to use a null pointer or reference an object that is not initialised, resulting in a crash or error message.
Array index out of bounds: This happens when a program tries to access an array element that is outside the valid range of indices, causing a crash or unexpected behavior.
Overflow or underflow: This occurs when a program tries to store a value that is too large or too small for the data type, causing a wrap-around or truncation error.
Memory leaks: This happens when a program fails to release allocated memory, causing it to consume more and more resources until the system becomes unstable.
Pro Tip:Â Always test your code thoroughly and use error-handling techniques like try-catch blocks to prevent runtime errors from crashing your program.
How to Identify Runtime Errors
Runtime errors can be tricky to identify, but there are a few common signs to look out for. These can include:
The program crashes or stops running unexpectedly.
An error message pops up on the screen with a description of the error.
The program runs but produces unexpected or incorrect output.
Sometimes the error message will contain clues as to what caused the error. For example, “division by zero” suggests that there is a problem with a certain calculation or formula.
One example of error coding might be if a programmer forgot to include a semicolon at the end of a line of code, which could result in a runtime error. It is important to carefully review and test your code to catch and fix any errors before running it in a live environment.
Logical Errors
Logical errors occur when a program runs without any error message but produces unintended or incorrect results. To detect these errors, programmers use error coding in their programs.
For example, consider a program that is designed to find the average of a series of numbers. Instead of adding the numbers and dividing by the total count, the programmer forgot to include the last number in the calculation. This is a logical error that can be detected by implementing an error code that would alert the user to this issue.
Error coding is fundamental to debug and troubleshoot programs, as it enables programmers to identify and resolve logical errors more efficiently. In addition, error coding helps users to determine precisely what caused a particular error and how to fix it.
Pro tip: When writing code, always include clear, concise error messages that help identify issues and provide solutions that are easily understood by the user.
Definition and Examples of Logical Errors
Logical errors are mistakes in computer programming that don’t cause the program to crash but produce incorrect results. An example of logical error coding can be seen in the following example:
Let’s say you’re writing a program to calculate the average of three numbers. Your code reads as follows:
int num1 = 5;
int num2 = 10;
int num3 = 15;
double average = num1 + num2 + num3 / 3;
You expect the average to be 10, but your code produces a result of 20. This is because the division operator has a higher precedence than the addition operator, and so the program is performing the division operation first before adding the three numbers. To get the correct result, you need to use parentheses to indicate the order of operations:
double average = (num1 + num2 + num3) / 3;
Pro tip:Â Always use parentheses to indicate the order of operations to avoid logical errors in your code.
How to Identify Logical Errors
Logical errors in coding can be difficult to identify and fix, but there are a few key strategies you can use to identify them effectively.
Here are a few examples of error coding:
Off-by-one errors: This type of error occurs when a loop or array goes one index too far or not far enough. For example, if an array has 10 elements, but a for loop goes up to 11, that’s an off-by-one error.
Divide by zero errors: This error occurs when a program tries to divide a number by zero.
Incorrect variable types: This error occurs when a variable is assigned the wrong data type. For example, if a string is assigned to an integer variable, this will result in an error.
Identifying logical errors often requires careful review of the code and tracking down the source of the problem. Using debugging tools and running test cases can also help pinpoint logical errors in your code.
Pro Tip:Â It’s essential to write clear and concise code, use testing and debugging tools, and run your program frequently to catch and fix logical errors as they arise.
Tools to Help With Error Coding
Error coding is a common issue encountered by software developers. It can be difficult to debug and detect, but luckily there are several tools you can use to help.
In this article, we will explore some of the ways that you can identify and fix errors in your code. We’ll discuss the benefits and drawbacks of various types of error coding tools, and then provide an example of a common error coding issue.
Integrated Development Environments (IDEs)
Integrated Development Environments (IDEs) are software applications that provide a suite of tools to help with error coding and software development.
An example of error coding can be a syntax error, such as a missing semicolon or a spelling mistake. IDEs can help identify these errors with real-time notifications, color-coding, and auto-completion features. Popular IDEs include Eclipse, Visual Studio, and IntelliJ IDEA
By using an IDE, developers can streamline the coding process, boost productivity, and catch errors before they become big problems. Pro tip: Before selecting an IDE, ensure that it supports the programming languages and features that you need.
Linters and Static Analysis Tools
Linters and static analysis tools are software programs that help developers identify and fix errors in their code before it causes any problems.
An example of error coding is a syntax error in which the code is written in a way that the computer cannot understand it. Linters and static analysis tools help detect syntax errors, as well as more complex errors like logical issues, type errors, and quality issues in the code.
Some popular linters and static analysis tools include ESLint for JavaScript, PyLint for Python, RuboCop for Ruby, and SonarLint for various programming languages.
By using these tools, developers can improve the overall quality of their code and reduce the likelihood of errors, security vulnerabilities, and performance issues. Pro Tip: Using linters and static analysis tools regularly can save developers a significant amount of time and effort in the long run.
Debugging Tools
Debugging tools are essential for identifying, diagnosing, and fixing errors in coding. These are the tools used by developers to debug their code quickly and efficiently. There are several debugging tools available, each specialising in different aspects of code debugging.
Some of the most commonly used debugging tools are:
- Debuggers: Debuggers are applications that allow developers to track the execution of a program line by line, set breakpoints, and examine variables’ values in real-time.
- Linters: Linters are tools that analyse source code, detect syntax errors, and provide suggestions for improvement.
- Profilers: Profilers are used to measure the performance of code, identify its bottlenecks, and optimise it for better performance.
- IDEs: Integrated development environments are software applications that provide a complete environment for coding, debugging, and testing.
Using these tools can help save countless hours of troubleshooting by providing a quick and clear solution to your coding problem.
Best Practices for Avoiding Error Coding
Error coding is a common problem that many developers and coders run into, and it is important to be aware of best practices to avoid potential errors. Error coding can lead to system crashes, inaccurate results, and wasted time.
This article will discuss some of the best practices to help you avoid potential error coding and make sure your code runs smoothly.
Establish Coding Standards
Establishing coding standards is crucial in software development to ensure that the code is consistent, maintainable, and free from errors.
Here are some best practices for avoiding error coding:
1. Consistency is key: Maintain a consistent naming convention, indentation style, and commenting format to make the code readable and understandable.
2. Keep it simple: Avoid overly complicated code that is difficult to follow. Use concise and straightforward syntax and avoid convoluted code structures.
3. Test early and test often: Use automated testing tools to test the code regularly and detect errors at an early stage. This will help in reducing the impact of errors on the overall product.
4. Refactor regularly: Refactor the code regularly to remove any redundancy or unnecessary code. This will improve the efficiency of the code and make it easier to maintain.
5. Document the code: Document the code using comments and clear documentation to help other developers understand your code and minimise errors.
By establishing coding standards, you can avoid errors in your code and ensure a more efficient, maintainable, and scalable software product.
Code Reviews
Code reviews are a crucial part of software development and help to prevent error coding. One example of error coding is when a line of code produces unexpected results or crashes the application.
Here are some best practices for avoiding error coding during code reviews:
- Write clear and concise code that follows standard conventions and is easy to read and understand.
- Use descriptive names for variables, functions, and classes.
- Test your code thoroughly before submitting it for review.
- Break down large blocks of code into smaller, more manageable chunks.
- Identify and remove redundant code.
- Use error handling to prevent crashes and unexpected behaviour.
- Encourage constructive feedback and collaboration among team members during code reviews.
Pro tip: It’s a good idea to have someone else review your code to catch errors and offer suggestions for improvement.
Testing and Debugging
Error coding can occur in programs or applications due to a variety of reasons such as syntax errors, semantic errors, runtime errors, or logical errors. Testing and debugging are crucial steps in resolving these errors before deployment, to ensure the functionality and reliability of the program.
Some of the best practices for avoiding error coding include:
1. Writing clean and organised code. This helps in identifying errors easily, and it also makes it easy to maintain the code.
2. Testing the code regularly, including unit testing, integration testing, and acceptance testing. This helps in identifying and fixing errors early on.
3. Using version control and deploying incremental updates, which minimises the risk of introducing new errors.
An example of error coding could be a function that accepts incorrect arguments, causing the program to crash or produce incorrect results.